Migration API Technical Reference
The Migration API endpoint allows developers to create and update documents in a Prismic repository.
The data format for the Migration API is described below. The format follows the response format from the Document API, though the Document API includes properties that are unnecessary for the Migration API. If you query a document object from the Document API and then send it to the Migration API, the Migration API will ignore the unnecessary properties and accept the rest.
Documents will be created as drafts, which you can publish in your repository.
By default, documents created or updated via the Migration API will be added to a migration release, accessible from the Migration Releases
tab in your repository. (To create your changes as drafts instead, add an inDocuments
property. See below.)
To include media (images, files, videos) in your documents, you will need to upload the media to your repository (either via the UI or the Asset API) and then fetch the ID for the media asset to include in your request to the Migration API.
We recommend saving the id
from the POST response for each document upon creation, as you will also require this id
to make updates to existing documents.
- Each request to the API can contain one document, which will be saved in the repository as a draft.
- Requests are limited to one per second.
This feature is in a closed beta
This feature is only available by request. Join the waitlist.
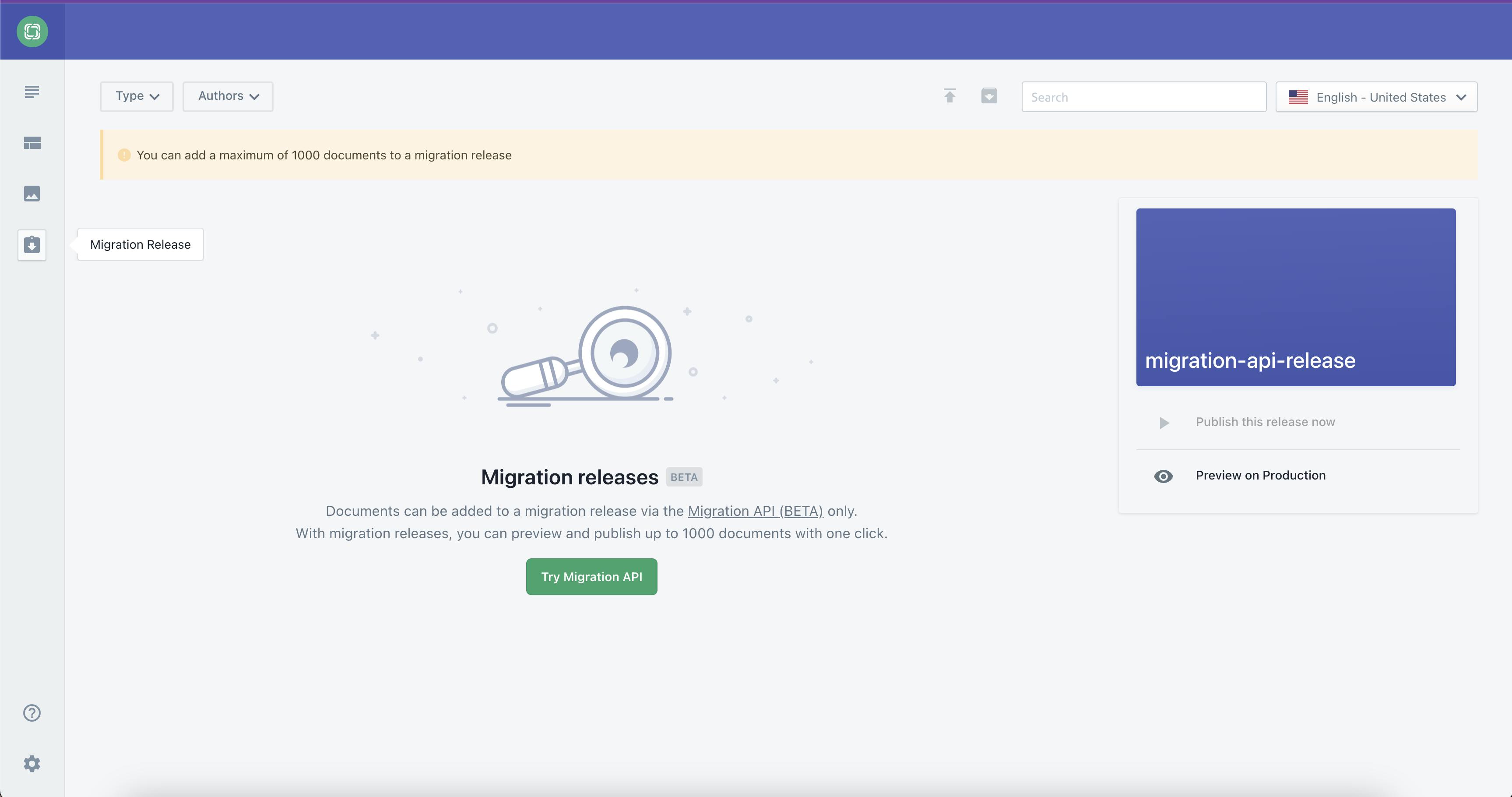
Admin users can try the Migration API using a demo key. Go to the Migration Releases tab in your repository for instructions.
{
"title": "Example Document",
"uid": "example-document",
"type": "example_type",
"lang": "en-US",
"tags": [
"Red",
"Yellow",
"Blue"
],
"alternate_language_id": "ZRFHsxIAAFYAsY--",
"data": {
"example_boolean": false,
"example_color": "#e0dbfd",
"example_date": "2024-12-23",
"example_timestamp": "2023-02-20T01:12:12+0100",
"example_integration": {
"id": "3"
},
"example_shopify_integration": {
"id": 7505689739510
},
"example_embed": {
"embed_url": "https://www.youtube.com/watch?v=S26CHpcD2zk"
},
"example_geopoint": {
"latitude": "-9",
"longitude": "-160"
},
"example_image_1": {
"id": "ZMgV5hAAAEADVsPS"
},
"example_image_2": {
"id": "ZMgV5hAAAEADVsPS",
"alt": "Example alt text",
"copyright": "Example copyright info",
"dimensions": {
"width": 800,
"height": 200
},
"edit": {
"x": 2,
"y": 28,
"zoom": 3,
"background": "#ffffff"
},
"mobile": {
"id": "ZMgV5hAAAEADVsPS",
"alt": "this is some alt text",
"copyright": "some copyright info"
}
},
"example_web_link": {
"link_type": "Web",
"url": "http://www.prismic.io"
},
"example_document_link": {
"link_type": "Document",
"id": "ZMI65RQAADkECUFM"
},
"example_media_link": {
"link_type": "Media",
"id": "ZMgV5hAAAEADVsPS"
},
"example_number": 23,
"example_select": "Apples",
"example_content_relationship": {
"link_type": "Document",
"id": "ZMI65RQAADkECUFM"
},
"example_group": [
{
"key_text_description": "Lorem",
"number_price": 55
},
{
"key_text_description": "Ipsum",
"number_price": 60
}
],
"example_rich_text": [
{
"type": "heading3",
"text": "This is a heading3",
"spans": []
},
{
"type": "paragraph",
"text": "Bold and italic example.",
"spans": [
{
"start": 0,
"end": 3,
"type": "strong"
},
{
"start": 8,
"end": 14,
"type": "em"
}
]
},
{
"type": "image",
"id": "ZPrkIhYAACcAYvyB",
"alt": "Example alt text",
"copyright": null,
"dimensions": {
"width": "512",
"height": "512"
}
},
{
"type": "embed",
"oembed": {
"embed_url": "https://prismic.io/"
}
}
],
"//": "The default slice zone is 'slices'",
"slices": [
{
"variation": "default",
"primary": {
"example_number": 11
},
"slice_type": "example_slice_type_1"
}
],
"example_slice_zone": [
{
"variation": "example_variation",
"items": [
{
"example_select": "Dog"
}
],
"slice_type": "example_slice_type_2"
}
]
}
}
The following code queries a document from the Document API, mutates that document, and then PUTs it back in the repository via the Migration API.
import { createClient } from '@prismicio/client';
import fetch from 'node-fetch';
async function init() {
const repository = 'example-repo';
const apiKey = 'put your demo key here';
const email = 'example_email@example.com';
const password = 'example-password';
// Fetch a document from your repository
const client = createClient(repository, { fetch });
const doc = await client.getFirst();
// Mutate the document
doc.data.example_key_text = 'This text will be updated.';
// Construct the request URL
const url = `https://migration.prismic.io/documents/${doc.id}`;
// Get an auth token
const authResponse = await fetch('https://auth.prismic.io/login', {
headers: {
'Content-Type': 'application/json',
},
method: 'POST',
body: JSON.stringify({
email,
password,
}),
});
const token = await authResponse.text();
// Send the update
const response = await fetch(url, {
headers: {
Authorization: `Bearer ${token}`,
'x-api-key': apiKey,
'Content-Type': 'application/json',
repository,
},
method: 'PUT',
body: JSON.stringify(doc),
});
console.log(await response.json());
}
init();
Authorization
A user session token from the Authentication API.
repository
The repository ID (e.g. your-repo-name
).
x-api-key
A key required for accessing the Migration API Beta.
https://migration.prismic.io/documents
The JSON body of every POST request must contain the following required properties:
title
type
uid
(if present on type)lang
data
https://migration.prismic.io/documents/:id/
:id
is the ID for an existing document.
The JSON body of every PUT request must contain the following required properties:
uid
(if present on type)data
The following properties will be ignored if they are included in the body:
title
type
lang
alternate_language_id
The body can also include a tags
property.
title
A display title to appear in the document list.
type
ID of the document’s type. Required for POST requests.
lang
The document’s locale.
data
An object containing the content of the document. Each key in the object should be a field or slice zone, and each value should be the content. (See the structure of the fields below.)
tags
An optional list of tags for the document. (Existing tags will be deleted if this property is omitted in a PUT request.)
uid
The document’s UID, which must be unique to that type. Required if present on the type and disallowed if absent on the type.
alternate_language_id
An optional ID for the document for which you are creating a translation.
inDocuments
A boolean that specifies whether documents should be created as drafts in the document list rather than in a migration release. (Default: false
)
The data
property represents the content of the document. Each key in the data object should be a field
or slice zone
on the document type, and the value should be the content.
The content of data
must conform to the structure of the document type.
If the field has a default value configured, the default value will be used for null values.
The string must be a hexadecimal color value.
Same as the value for a link with a link_type
of "Document"
.
The string must match the format YYYY-MM-DD
.
The object can optionally contain an embed_url
property with a URL as its value. Other properties will be ignored.
An empty object or an object with latitude
and longitude
properties, each with a value string representing a number. The value of latitude
must be between -90 and 90. The value of longitude
must be between -180 and 180.
An array of field objects.
An object with the required property id
and optional properties alt
, copyright
, dimensions
, edit
, x
, y
, zoom
, and background
.
id
string (required)
A string that exactly matches the ID of an exiting image in Prismic’s media library.
alt
string
The alt text for the image.
copyright
string
Copyright information for the image.
dimensions
object
An object with optional width
and height
properties, with numbers for their values, defining the image resize. If thumbnail width or height is already configured in the type, this value will be ignored.
edit
object
Parameters for editing the image. The object can contain:
x
: X coordinate for crop as an integer
y
: Y coordinate for crop as an integer
zoom
: Zoom level as an integer
background
: Background color as a hexadecimal code (defaults to transparent for PNGs and #ffffff
for other file types)
The image object can also contain thumbnail properties. The key should be the name of the thumbnail and the value should be an object with properties as described in the table above.
The ID of an item from your integration.
For shopify integration, the ID comes from your Shopify catalog. See the integration docs for more information.
A string of text.
An object with the required property link_type
and conditional properties depending on the type.
link_type
string (required)
One of "Document"
, "Web"
, or "Media"
.
id
string
Required if link_type
is "Document"
or "Media"
.
For "Document"
, id
must be the ID of any document in the repository, published or unpublished.
For "Media"
, id
must be the ID of an item from the Media Library.
url
string
Required if link_type
is "Web"
.
An absolute URL.
Value must conform to max
and min
if either is defined in the type.
An array in which each object represents a block element.
A rich text field must contain an array of content blocks. Every block must have a type
property with one of the following values:
"paragraph"
"o-list-item"
"list-item"
"heading1"
"heading2"
"heading3"
"heading4"
"heading5"
"heading6"
"preformatted"
"embed"
"image"
Every block except for those with a type of embed or image can have the following properties:
text
string (required)
The text content of the block.
spans
array
An array of objects describing inline markup. Each object must have a type with the value of either "em"
, "strong"
, or "hyperlink"
. Each object must also have start
and end
properties denoting the starting and ending indexes of the span. Spans can overlap. Spans of type hyperlink
must also have the properties defined for a link field.
direction
string
Value can only be "rtl"
, which defines the text as right-to-left.
oembed
object
Required only for blocks with a type
of "embed"
. The object should contain an embed_url
property with an absolute URL as the value.
Blocks with a type of "image"
must have the properties defined for the image field.
If value is a string, the string must match one of the options defined in the type. If the field has a default value configured, it will be used for null values.
Each slice zone property must be an array of zero or more slice objects. By default, all types in Prismic have a slice zone property called slices
, but the developer can change the name of the slice zone and add other slice zones.
Each slice object must have the following properties:
slice_type
string (required)
The ID of the slice type (e.g. "hero_image"
).
variation
string (required)
Must match one of the variations defined in the configuration for the slice type, even if there is only one variation. The default variation for slice types is called default
.
slice_id
string
A optional string in the following format: slice_type
+ $
+ uuid v4
. Must be unique within the document. If omitted, it will be generated automatically.
slice_label
string
A label that will appear in the Document API response. If omitted, it will be set to null
.
primary
object
An object containing the fields of the non-repeatable zone of the slice.
items
array
An array of objects containing the fields of the repeatable zone of the slice.
Was this article helpful?
Can't find what you're looking for? Spot an error in the documentation? Get in touch with us on our Community Forum or using the feedback form above.